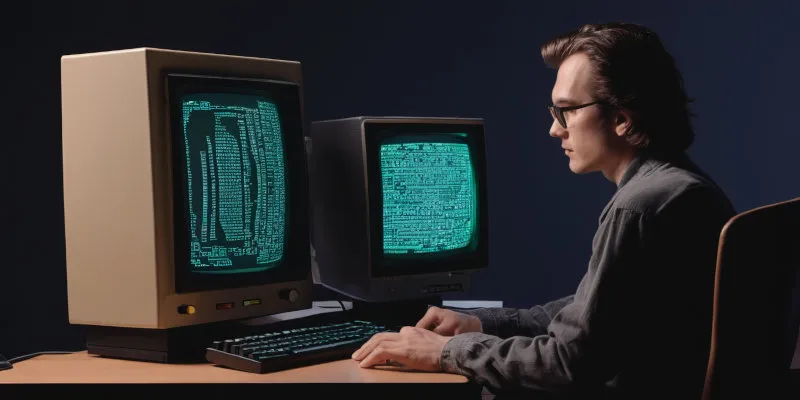
How to write your first Playwright test
July 4, 2024About 1 min
Prerequisites
To write and run your first playwright test you need Node.js to be installed on your System, the most easy way is using a manager like Volta.
# install Volta
curl https://get.volta.sh | bash
# install Node
volta install node
Install Playwright
npm
npm init playwright@latest
pnpm
pnpm create playwright
yarn
yarn create playwright
Run the install command and choose intallation options to get started:
- Choose if you want to use TypeScript or JavaScript
- Select the name of the Tests folder (default is tests or e2e if you already have a tests folder in your project)
- Add a GitHub Actions workflow
- Install Playwright browsers
After completing the init script execution playwright will create an example project containing some files:
playwright.config.ts
package.json
package-lock.json
tests/
example.spec.ts
tests-examples/
demo-todo-app.spec.ts
Execute example tests
By default Playwright example tests runs on three browsers: Chromium, Firefox, and Webkit, utilizing three parallel workers. Tests operate in a headless mode, preventing browser windows from opening. Test outcomes and logs are displayed in the terminal.
npm
npx playwright test
pnpm
pnpm exec playwright test
yarn
yarn playwright test
Let's now analyse a test to understand how it works:
// Import test and expect functions from playwright module,
// in order to declare a test and perform assertions
import { test, expect } from '@playwright/test';
// Invoke the test function, the first argument is the test name,
// the second one is an asyncronous function containing all tests actions,
// the asyncronous function can use built-in fixtures, here we're using `page`
test('has title', async ({ page }) => {
// Invoke the goto method of the page fixture to navigate a URL
await page.goto('https://playwright.dev/');
// Expect a title "to contain" a substring.
await expect(page).toHaveTitle(/Playwright/);
});
Show test report
npm
npx playwright show-report
pnpm
pnpm exec playwright show-report
yarn
yarn playwright show-report